Bluetooth LE and Hybrid Applications
Can Bluetooth LE and Hybrid Applications exist together? Yes! In this article, we provide an example of a hybrid application and Bluetooth Low Energy service, starting with the description of what a hybrid application is, then ending with the complete example.
Let's start by providing some definitions.
What is a hybrid application?
A hybrid application is a software that combines elements of both web applications and native apps. It is simply a web app that you put into a native app shell. You can download it from the app store (Google Play for Android devices or App Store for iOS devices), like a common native app. Once you have downloaded it from the store and you have installed it locally, the shell is able to connect to all the features the mobile platform provides through an embedded browser in the app (here you can start to understand why Bluetooth LE and hybrid applications can live together).
The browser and its plugins run on the backend, so the end user cannot see the entire architecture. Hybrid applications are very popular due to their versatility and ease of use, especially for those applications that do not require too many low-level operating system features. Usually, you write hybrid applications in HTML5, CSS and JavaScript and they run code inside a container.
Here is provided a list of three pros and three cons of developing a hybrid app.
Pros
- Build time of hybrid applications is very low compared to native apps;
- The same code operates on different platforms;
- They are cheaper to develop compared to building different versions of a native app for different platforms;
Cons
- The development team does not make experience developing for different platforms, but it works only with one framework;
- User Experience (UX) could be worse compared to a native app if the User Interface (UI) is not similar;
- The appearance of an application could be different between two platforms;
In another article, we have described why hybrid applications are very important. You can find the article here.
What is Bluetooth LE?
Bluetooth Low Energy (abbreviated with the acronym BLE) is a particular type of standard Bluetooth that, as the name says, has a reduced energy consumption. As the standard Bluetooth, it uses the same radio frequency in the 2.4 GHz free band in order to connect nearby devices together. The bit rate of Bluetooth LE is 1 Mbps (with an option of 2 Mbps in the BLE 5) and the maximum transmission power is 10 mW (100 mW for BLE 5). This means that the usage power of Bluetooth LE is less than half compared to standard Bluetooth. The image below is an overview of Bluetooth LE technology.
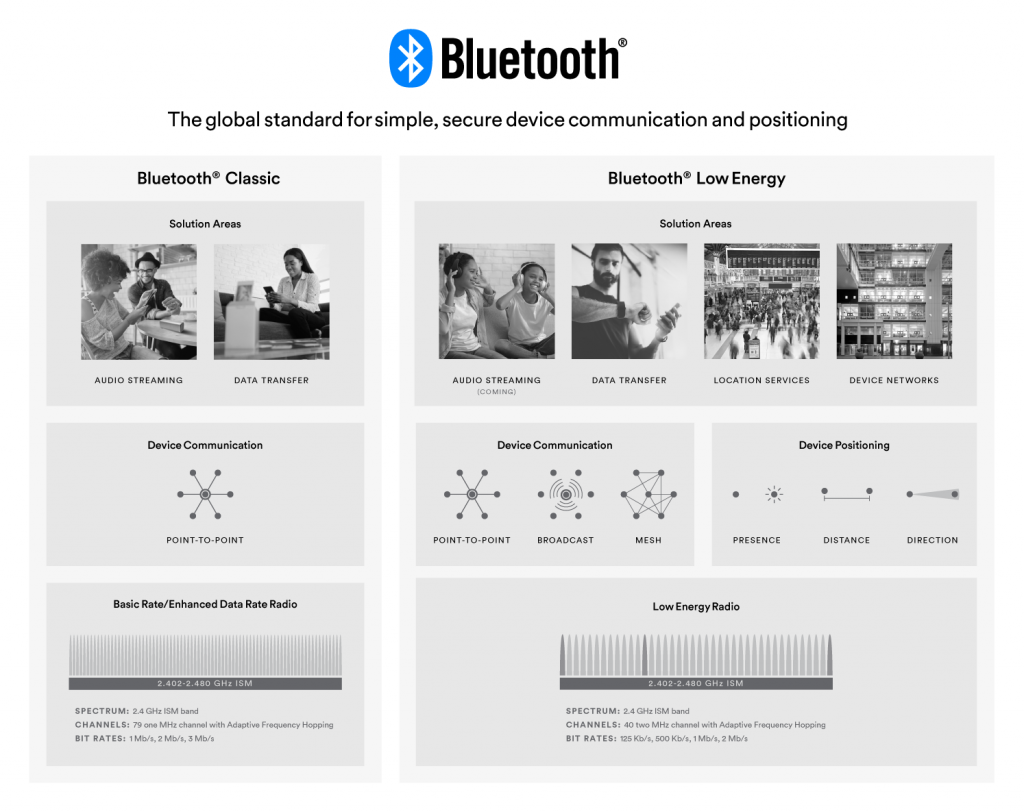
Bluetooth Technology Overview Graphic
Here, you can find more information about the difference between standard and low-energy Bluetooth. As for hybrid applications above, here we provide three pros and three cons.
Pros
- Bluetooth LE offers very low power consumption and hence devices battery life can be very long (this is due to the fact that both master and slave devices can go to deep sleep mode between their transactions. Master device wakes up the slave device when it has to start a transaction);
- It can be used for small-size data transfer, especially in IoT (Internet of Things) based applications (up to 255 bytes of message capacity);
- Connection establishment procedure and data transfer are very fast. It takes about 3 ms;
Cons
- It can not be used for long-distance wireless communications, unlike cellular and wifi devices (it supports up to 200 meters in line of sight);
- Bluetooth LE is open to attack and interception due to wireless transmission/reception (without authentication layers);
- It can not be used for higher data rates as offered by wifi and cellular technologies. It supports 1 Mbps and 2 Mbps data rates;
Bluetooth LE and hybrid applications: an example
Two of the most popular options to develop hybrid mobile apps are Ionic and Xamarin frameworks. From the Ionic vs. Xamarin page on Ionic site, "Ionic is a platform that uses widely-known, standards-based web technologies and languages to create award-winning mobile experiences, and is built on the broader web and JavaScript ecosystem. Xamarin is a Microsoft product and embraces the Microsoft ecosystem".
In this example, we use Ionic framework with Cordova (which was PhoneGap in the past), because the plugin we need is a Cordova one (https://github.com/randdusing/cordova-plugin-bluetoothle).
Prerequisites
To create the example app, you must have some prerequisites:
- Ionic installed on your machine (official installation guide);
- Visual Studio Code (or an IDE of your choice);
Step 1: Creation of the app
As the first step, we have to create the app using ionic cli (command line interface). We choose a tabs type of Ionic app with Angular framework. Let's create the app with the following command:
ionic start HybridBluetoothLE tabs --cordova --type=ionic-angular
where we specify the name of the project (HybridBluetoothLE), the type of Ionic app (tabs), the runtime of the app (Cordova), and the type of framework used to develop the app (ionic-angular).
Now, we have the first skeleton of the app that we want to transform into a Bluetooth LE scanning device application. Let's go ahead to integrate the Bluetooth LE Cordova plugin we need.
Step 2: Integration of the Bluetooth LE Cordova plugin
In this moment we can add the Bluetooth LE plugin with the following command:
ionic cordova plugin add cordova-plugin-bluetoothle
and also add it to package.json file with:
npm install @awesome-cordova-plugins/bluetooth-le
If all steps are completed without any trouble, we can go ahead to start using this plugin.
Step 3: Creation of scan page
First of all, we have to create the scan devices page, in order to see our plugin in action. In this page, we create two buttons: the first one to start the devices scanning and the second one to stop the scan. We must be sure that the mobile device has the location enabled and the app has the location permissions enabled. To do this, for the iOS platform, we have to add into the config.xml file the following permissions settings under the iOS platform tag:
<config-file parent="NSBluetoothAlwaysUsageDescription" target="*-Info.plist"> <string>Our app uses bluetooth to find, connect and transfer data between different devices</string> </config-file> <config-file parent="NSBluetoothPeripheralUsageDescription" target="*-Info.plist"> <string>The app uses bluetooth to find, connect and transfer data between different devices</string> </config-file> <config-file parent="UIBackgroundModes" target="*-Info.plist"> <array> <string>bluetooth-central</string> <string>bluetooth-peripheral</string> </array> </config-file>
Once this is done, we can start to create the logic behind Bluetooth LE in a hybrid application. First of all, we have to initialize the central device (the device that starts the communication) with the following function:
this.bluetoothle.initialize(this.CENTRAL_PARAMS).subscribe((central) => { console.log("central status ", central); if (central.status === "enabled") { console.log("BLE is enabled"); } else { console.log("BLE is disabled"); } }, err => { console.log("initCentral ERROR: ", err) } );
after that, we can really start/stop the device scan with the following functions:
public startScan(){ let params: ScanParams = {}; this.bluetoothle.startScan(params).subscribe(device => { if(device.name){ console.log(device); this.scannedDevices.push(device); } }, error => { console.log(error); }); } public async stopScan(){ await this.bluetoothle.stopScan(); }
and put all the scanned devices into an array called scannedDevice. This array contains all the information of every single device, such as the connection address. It is the string we need to connect to the selected device.
Step 4: Connect/disconnect device
Now, in the second tab we have to create a page where we can select a device and connect us to. In order to do that, we must use the following function:
public async connect(){ let connection_params = { "address": this.dataService.selectedDevice.address, "autoConnect": false, "clearCache": true } let wasConnected = await this.bluetoothle.wasConnected(connection_params); if(wasConnected.wasConnected){ await this.bluetoothle.disconnect(connection_params); } this.bluetoothle.connect(connection_params).subscribe(async success => { console.log("connection - BLE Connect: ", success); this.connected = success.status == "connected"; }, async error => { await this.bluetoothle.disconnect(connection_params); }); }
Before create a new connection with the selected device, you have to be sure that you are not connected to it. To do this, you can previously disconnect it with the built-in disconnection function:
public async disconnect(){ let connection_params = { "address": this.dataService.selectedDevice.address, "autoConnect": false, "clearCache": true } this.bluetoothle.disconnect(connection_params).then(success => { this.connected = false; }).catch(error => error); }
Conclusions
Now, we are able to create a Hybrid Application that allows us to scan and connect us to Bluetooth LE peripherals. In this small tutorial, we have seen that a low-level service such as Bluetooth can be used in a hybrid application using a few simple steps.